Put data
PUT API use to update data.
Send only key-value pairs which you want to update in request body. Request url contains 'ID' of the data object.
Update single key
Update single key in single object.
Request body contains object of key-value pair.
Request body
Generated url:
Here we have to pass primary key number (mostly its 'id') of the object in the request url.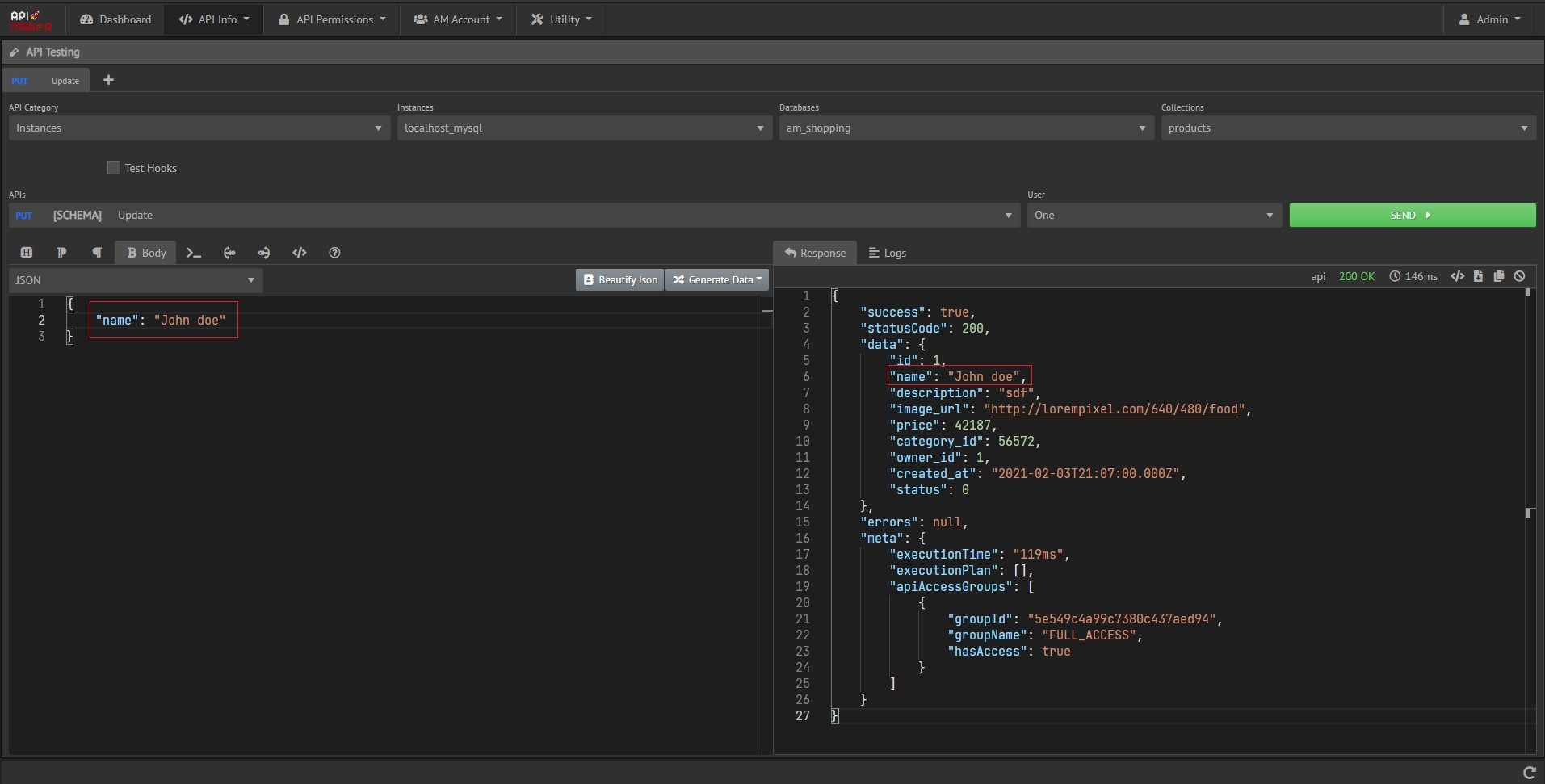
Update multiple keys
Update multiple keys in single object.
Request body contains object of key-value pairs.
Request body
Generated url:
Here we have to pass primary key number (mostly its 'id') of the object in the request url.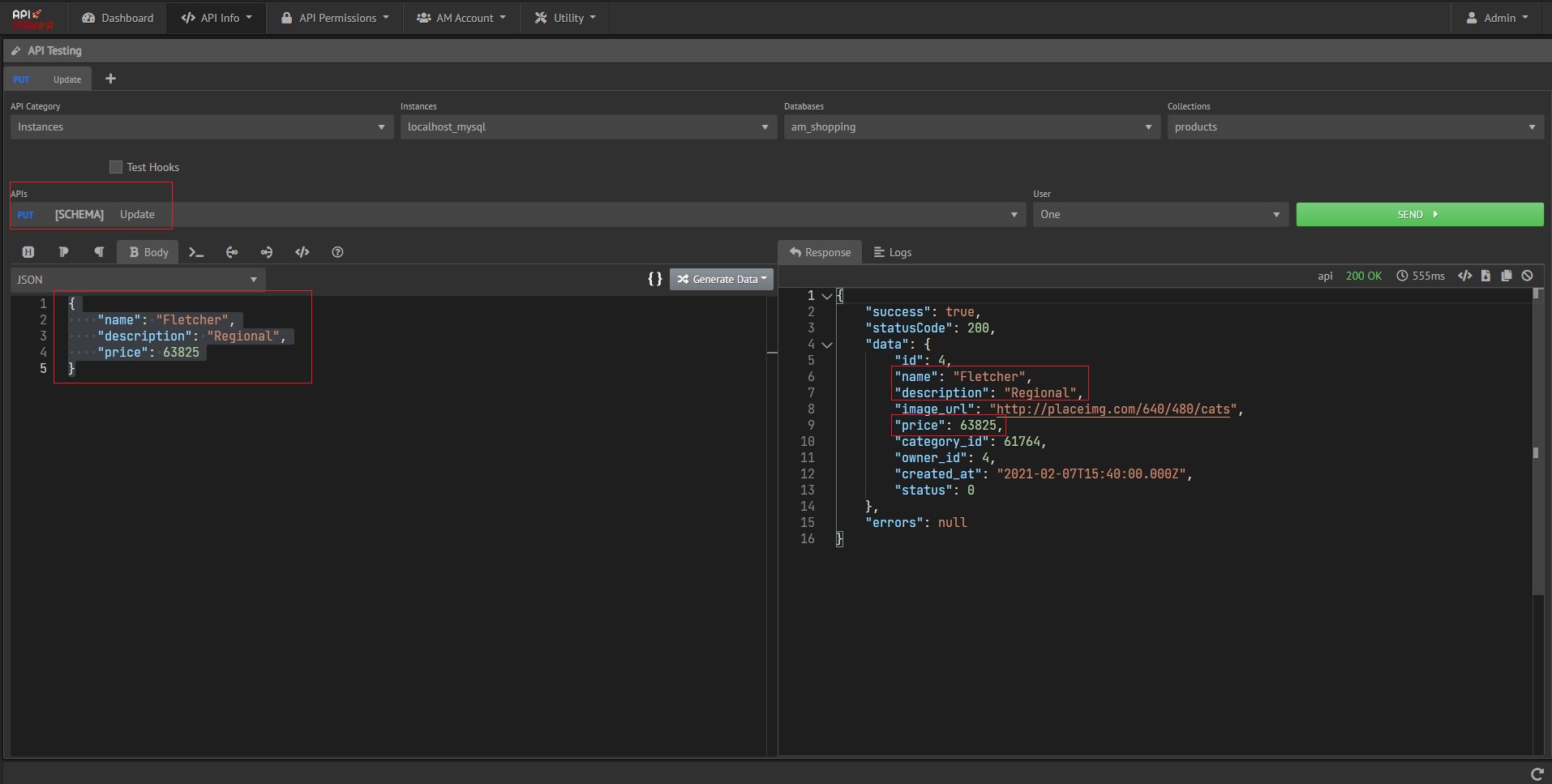
Custom PrimaryKey
Find data using custom PrimaryKey.
Here in products collection we have 'id' is the primary key, but we can find data using making any column as primary key.
If we do not pass custom primary key, system will take default primary key.
Request body
Generated url:
In above generated url 'owner_id' is the given custom primaryKey.Select
While an update, we can set query param 'select' to get specific keys in response.
Request body
Generated url:
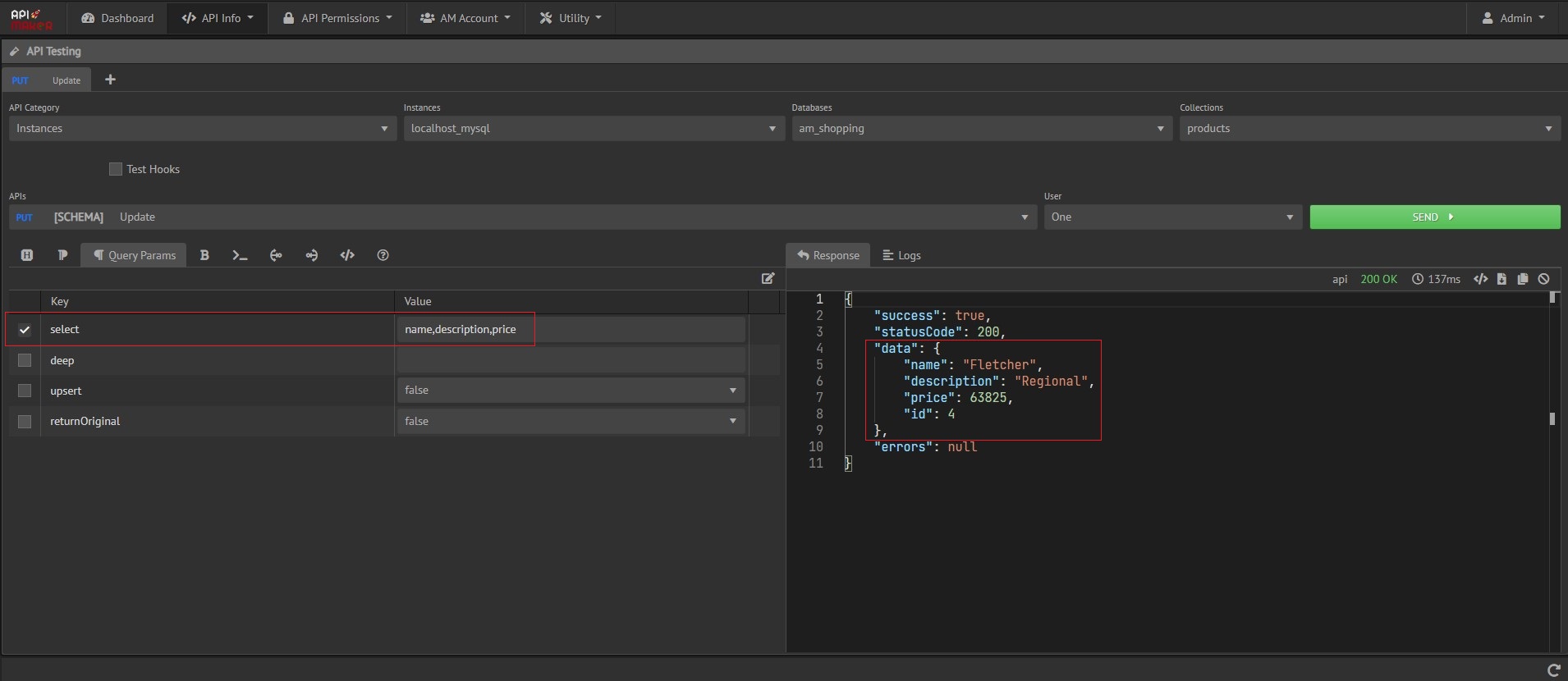
Deep
While an update, we can set query param 'deep' to get inherit data.
Request body
Generated url:
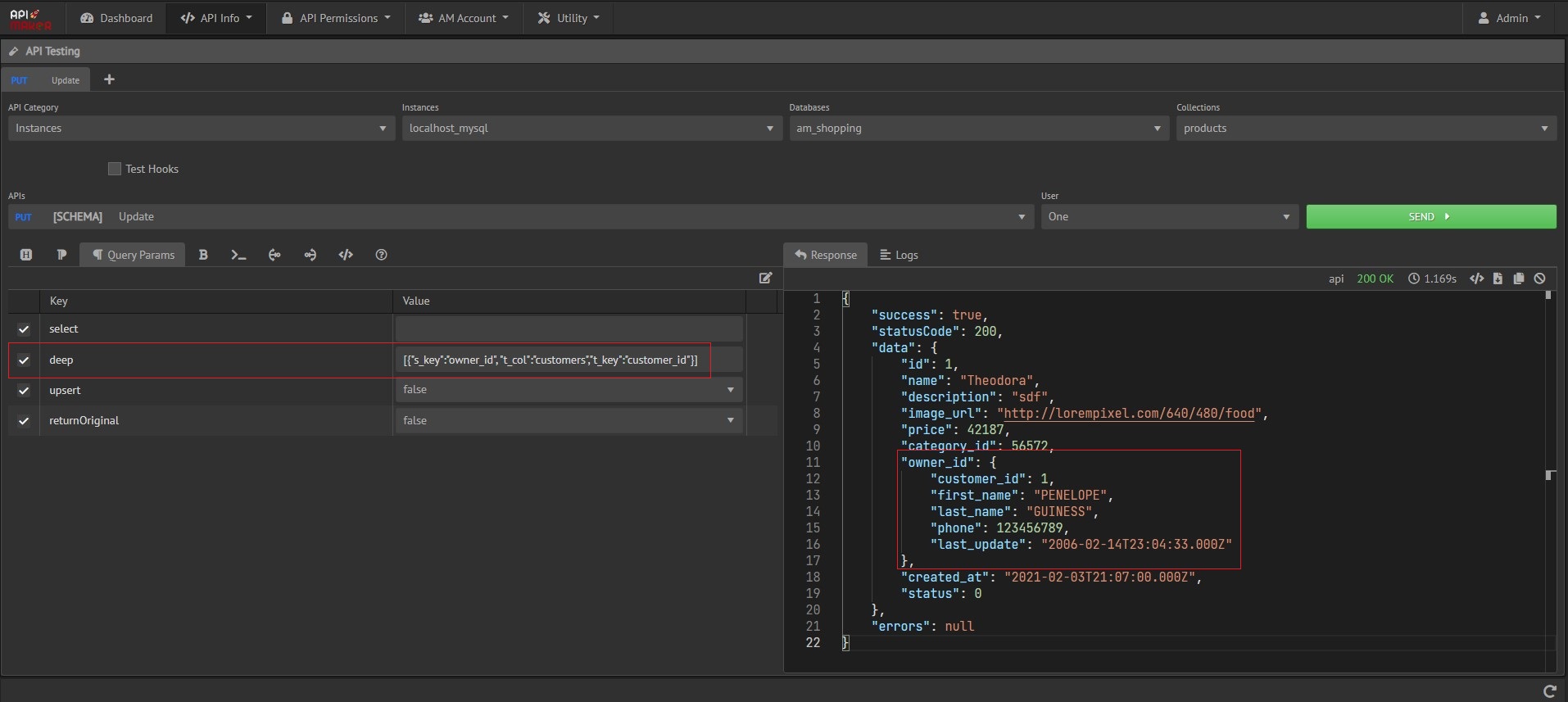
Upsert:true
If upsert set true, and the request data not found system will add requested data in a database.
Here we try to update data which has customer_id=101
, system cannot find it, and the upsert set true, so it saves that object.
Request body
{
"first_name": "Sophia",
"last_name": "Molly",
"phone": "9762611635",
"last_update": "2021-07-25T06:45:45.185Z"
}
Generated url:
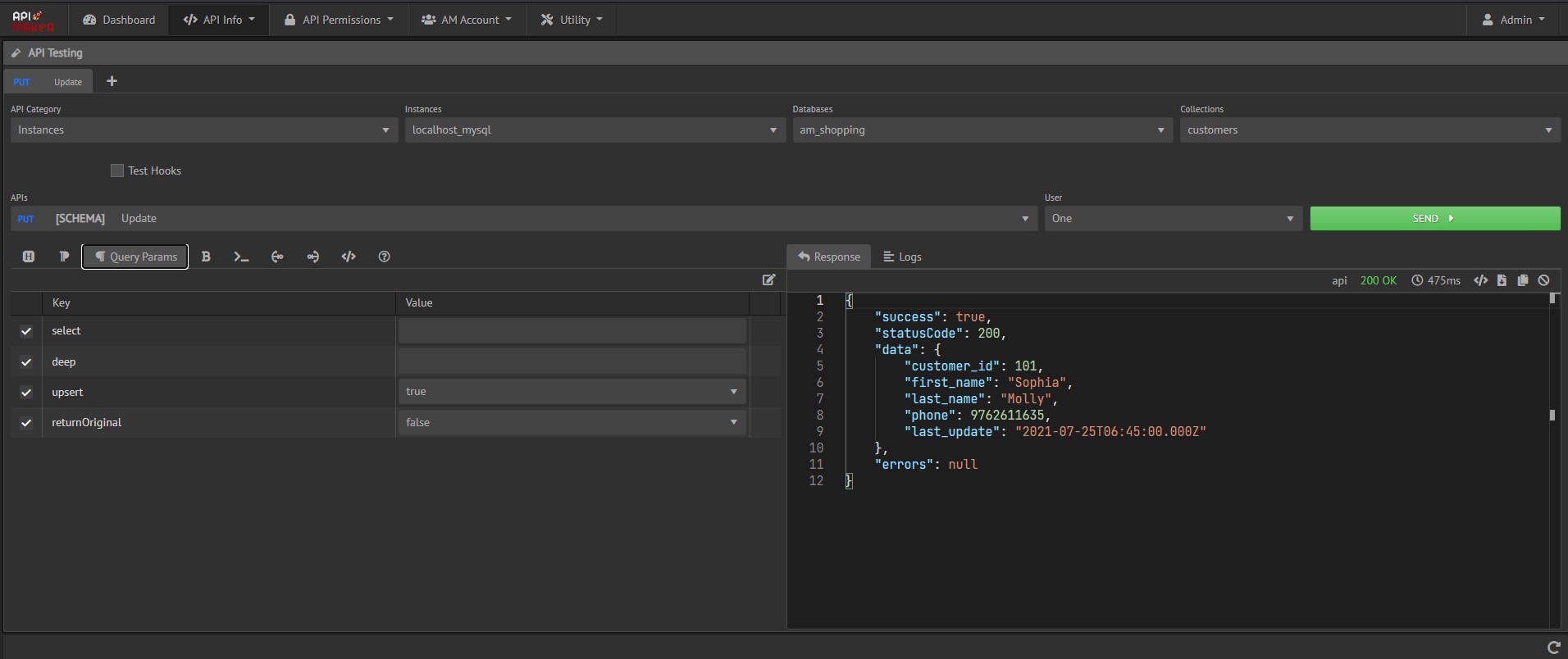